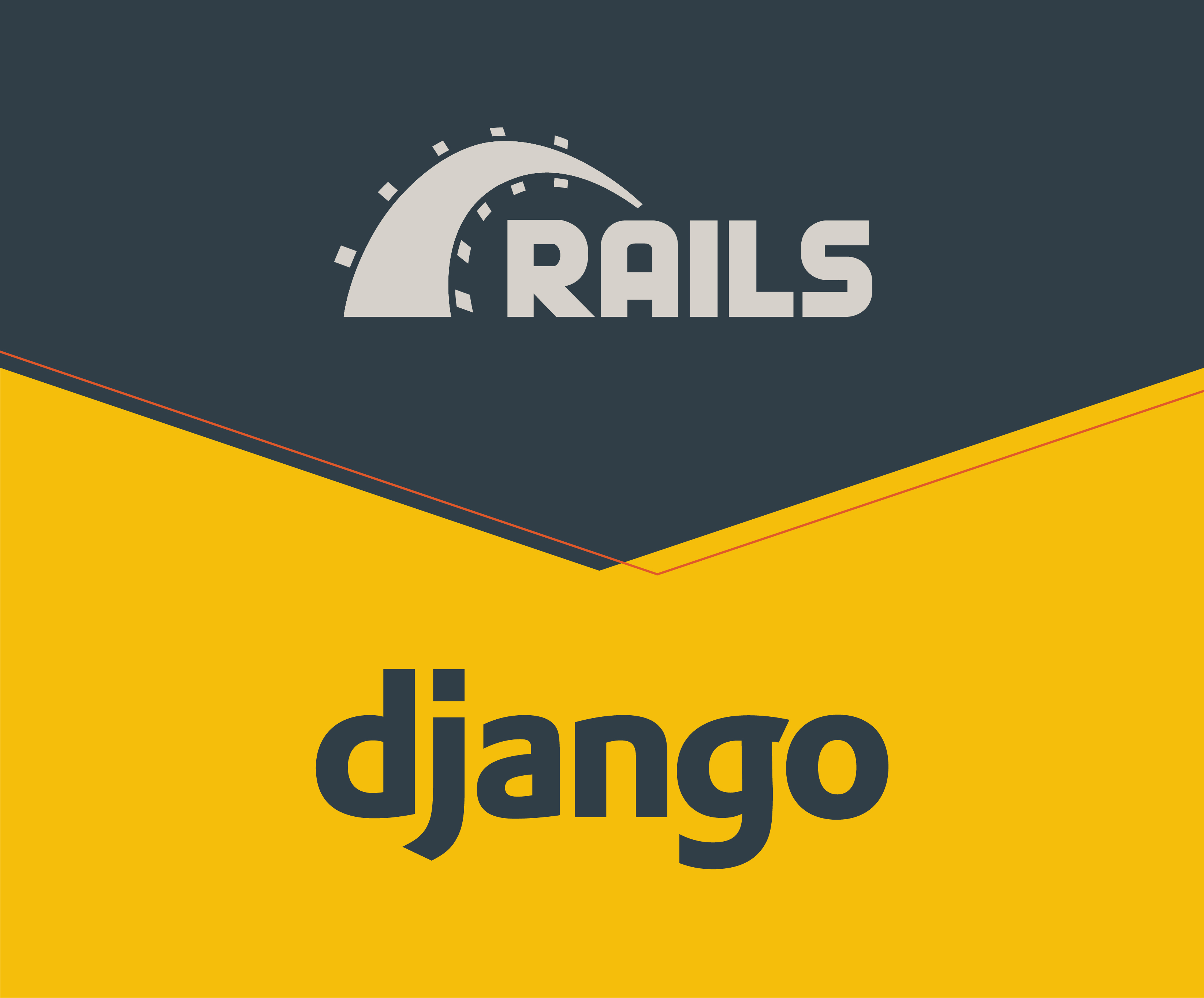
After almost two years of working on a project with a Ruby on Rails(RoR) backend, I switched to a project with a Django backend last Spring. In the beginning, I was optimistic about the large number of similarities between the two frameworks and thought that it would be a seamless transition. Python and Ruby seemed similar enough that I was fairly certain I would enjoy working with Django as much as I enjoy RoR.
Now it has been roughly five months since I began working with Django and I have found a few areas where I feel that one framework has a clear advantage over the other. While there are some features of Rails that I am missing, I’ve found that Django has other impressive characteristics.
Ruby Syntax
Personally, writing in Ruby syntax feels more graceful than Python. Python has some syntax rules that are more restrictive than Ruby. I occasionally find myself slipping back into writing my code in Ruby by accident because the two languages have a lot of similarities. For example, here is the same method written in Ruby vs Python:
Ruby:
def calculate_tax(price)
if price > 100
price * 0.09
elsif price > 50
price * 0.07
else
price * 0.05
end
end
puts calculate_tax(75)
Python:
def calculate_tax(price):
if price > 100:
return price * 0.09
elif price > 50:
return price * 0.07
else:
return price * 0.05
print(calculate_tax(75))
As you can see, there is plenty of overlap between the two implementations. However, even after five months, I still find myself forgetting to add parenthesis to the end of a method call or a colon to the end of a conditional statement. As far as readability, seeing the “end” keyword helps me to more quickly find where the method or conditional statement is over. In contrast, the abrupt end of Python methods and statements was an adjustment.
Some keywords have been more helpful to me than others in syntax. After working in Ruby for a while, I have come to think of the “return” keyword as unnecessary since Ruby just returns whatever value was last calculated. Ruby also does not care about indentation and spacing as much as Python does. This means that I can write code in Ruby without worrying about if the format is correct for it to compile and run and then clean my code up by running a lint command before committing. I am not afforded the same freedom and efficiency in Python.
Rails Console & Scaffolding
I find that the default Rails console is more user friendly than the Python shell. However, the main selling point of the Rails console is the scaffolding capabilities (AKA dynamically generated files and code templates). After getting spoiled by all the Rails magic that happens in development with scaffolding, Django feels more old fashioned, slow and like I am spending more time writing code that would have been generated automatically by Rails.
Active Record
RoR has my favorite ORM (Object-Relational Mapping) of all the web frameworks I have used. It was much faster for me to define models with complex relationships in RoR when compared to Django. This is thanks to RoR’s Active Record which provides each class that inherits it with robust query methods and data manipulation capabilities.
What I Like About Django
Installation
Django was much faster and more straightforward to install. Just a few short and simple steps and I was ready to start developing in Django. On the other hand, Ruby on Rails installation was much longer with more background knowledge required to get started. Having to learn to use bundle and gems to get RoR up and running was objectively more complicated than Django’s quick installation.
Automatic Migration Generation
There is one area that Django seems to have more magic than RoR. Django’s ability to detect changes in models and automatically generate a migration to perform those changes in the database has saved me a lot of time and effort. In RoR, I would have to generate a migration file that was basically empty and then fill it in the required operations to the database.
Structure
The project I am working on uses the Django REST framework which is a toolkit that provides a fast and efficient way to build RESTful APIs. This framework seems to provide more structure for developers than RoR did. There is less room for creativity and therefore the code seems to be more black and white as far as how to go about implementation. RoR implementation can be much more varied, which probably adds to its appeal for some people. However, I find that it is simpler to have a single path to the correct implementation than many flexible ways of writing the same functionality.
Conclusion
Ruby on Rails and Django both have their strengths in different departments. In my opinion, development in RoR is more smooth and efficient when compared with Django. Although there are a few categories that I feel Django excels in, I still have a preference for the “Rails way” of doing some things. Maybe that will change over time as I continue to advance my understanding of Django. Due to the similarities between the two frameworks, my experience with RoR was a great foundation for learning Django that I’m excited to continue building onto.
Loved the article? Hated it? Didn’t even read it?
We’d love to hear from you.
Great comparison between the two, really helpful. It is a good read.
I’m thinking about making this migration. Ruby on Rails is unfortunately becoming a very “tech bro” stack, and in my experience in the companies I’ve worked for this translates into a cult of “productivity” and “competence and excellence” that has done me a lot of harm. There seems to be more room for a healthier culture on the Python side. However, I feel that Rails really is a more mature technology. I’m willing to take this downside if it translates into a better work culture.